In modern software development, event-driven architectures (EDA) have gained immense popularity for their ability to create scalable, flexible, and resilient systems. One of the key challenges in building such systems is maintaining a decoupled communication model between different components. AWS EventBridge is a serverless event bus service that plays a crucial role in achieving this decoupling by facilitating the seamless transfer of events between services in real-time.
Introduction
This guide will explore how to leverage AWS EventBridge to build event-driven systems that are scalable, fault-tolerant, and easy to manage. We will walk through the fundamental concepts, demonstrate technical implementation, and highlight best practices for optimizing performance and security.
Background
Event-driven architectures have been around for decades, but the increasing complexity of microservices, distributed systems, and cloud computing has pushed event-driven paradigms to the forefront of modern software architecture. Event-driven systems allow components to communicate asynchronously through events, decoupling the producers of events (senders) from the consumers (receivers). This decoupling enhances flexibility, scalability, and fault tolerance.
AWS EventBridge is a fully managed event bus service that helps to simplify event-driven applications by providing the infrastructure needed to publish, process, and route events. Originally introduced as Amazon CloudWatch Events in 2016, EventBridge was rebranded in 2019 and expanded with new features, including better integration with third-party services and support for custom event sources.
Key Concepts
To effectively use AWS EventBridge, it’s important to understand the following key concepts:
- Event Bus: An event bus is a channel that receives events from event producers and routes them to one or more event consumers. EventBridge provides both default and custom event buses.
- Event Source: This is the component that generates events. In the context of EventBridge, it could be an AWS service (e.g., EC2, S3) or a custom application.
- Event Rule: Rules define the criteria for event matching. An event rule filters incoming events based on event patterns and routes the matching events to specific targets such as Lambda functions, SNS topics, or SQS queues.
- Event Target: Targets are the services or resources that will process events once they match a rule. Targets can be AWS Lambda functions, Step Functions, SQS queues, SNS topics, or even HTTP endpoints.
- Event Pattern: Event patterns are used to filter events. They define which events a rule will match based on specific event attributes (e.g., source, detail-type, etc.).
- Schema Registry: AWS EventBridge includes a schema registry to store and discover the structure of events, making it easier for consumers to handle events correctly.
Technical Implementation
High-Level Architecture
At a high level, an EventBridge-based event-driven system consists of the following components:
- Event Producers: These are the sources of events. These could be AWS services (like Lambda, S3, or DynamoDB) or custom applications that push events to EventBridge.
- Event Bus: The event bus receives events from the producers and holds them temporarily before they are routed to appropriate consumers.
- Event Rules: EventBridge allows users to define event rules that filter events based on their content and then forward matching events to one or more targets.
- Event Consumers: These are the services that process events, such as AWS Lambda functions, Step Functions, or even external systems connected via HTTP.
Here’s a high-level flow of how the components work together:
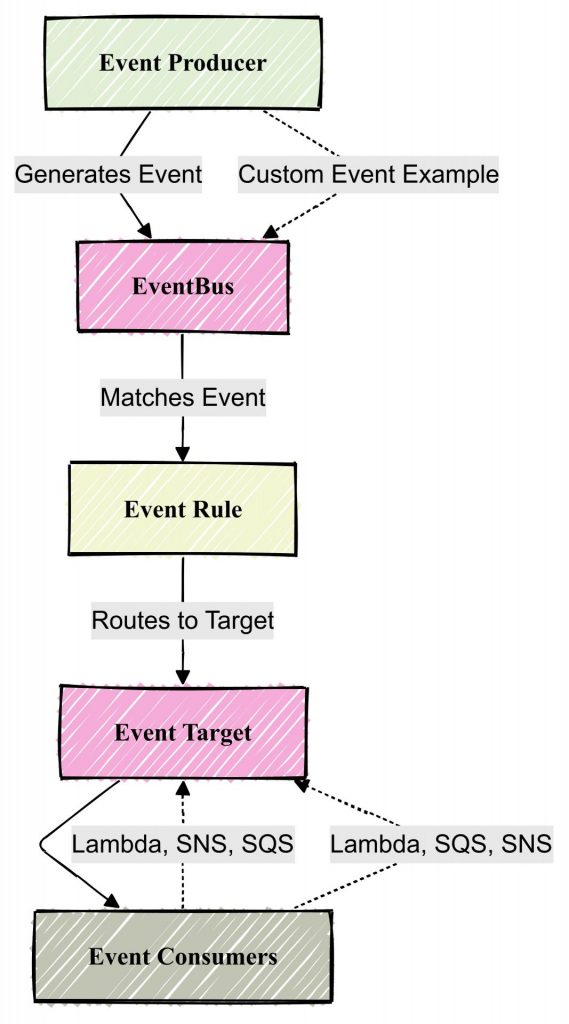
Step-by-Step Implementation
1. Create an Event Bus
First, you need to create an event bus in EventBridge. EventBridge provides a default event bus, but you can also create a custom one to handle specific events for your application.
CLI Command:
aws events create-event-bus --name my-custom-event-bus
Console:
- Navigate to the EventBridge console.
- Choose Create event bus and provide a name for your event bus.
2. Define an Event Rule
Once the event bus is created, you can define an event rule that matches specific events and routes them to the appropriate targets.
CLI Command:
aws events put-rule --name my-event-rule \
--event-bus-name my-custom-event-bus \
--event-pattern '{"source": ["my.custom.application"]}' \
--state ENABLED
Console:
- Go to the EventBridge console.
- Under Rules, choose Create rule.
- Select Custom event bus, and choose the event bus you created.
- Define the event pattern, such as
{"source": ["my.custom.application"]}
, and enable the rule.
3. Set Up Event Targets
Next, you need to configure the targets for the events that match your rule. EventBridge supports a wide range of targets, such as Lambda functions, SNS topics, and SQS queues.
CLI Command:
aws events put-targets --rule my-event-rule \
--event-bus-name my-custom-event-bus \
--targets '[
{
"Id": "1",
"Arn": "arn:aws:lambda:REGION:ACCOUNT_ID:function:my-lambda-function"
},
{
"Id": "2",
"Arn": "arn:aws:sqs:REGION:ACCOUNT_ID:my-sqs-queue"
}
]'
Console:
- In the Targets section of your rule, select Lambda function (or other targets like SQS, SNS).
- Choose the appropriate Lambda function or resource that should process the event.
4. Publish Events
Now, you can publish events to your EventBus. For example, your application can send custom events to EventBridge using the following CLI command.
CLI Command:
aws events put-events --entries '[{
"Source": "my.custom.application",
"DetailType": "Custom Event",
"Detail": "{\"key1\":\"value1\",\"key2\":\"value2\"}",
"EventBusName": "my-custom-event-bus"
}]'
Code Example (Python):
import boto3
import json
client = boto3.client('events')
def publish_event():
event = {
'Entries': [{
'Source': 'my.custom.application',
'DetailType': 'Custom Event',
'Detail': json.dumps({'key1': 'value1', 'key2': 'value2'}),
'EventBusName': 'my-custom-event-bus'
}]
}
response = client.put_events(**event)
print(response)
5. Event Processing
When the event matches the rule, it will be routed to the specified targets (e.g., Lambda function, SQS queue). Ensure that your Lambda function has the necessary permissions to process events.
Lambda Function (Python Example):
def lambda_handler(event, context):
print("Received event:", event)
# Process the event
return {"status": "success"}
Best Practices
1. Avoid Tight Coupling
One of the main benefits of EventBridge is decoupling. Ensure that your event producers and consumers are not tightly coupled. The event format should be simple and easily extensible.
2. Use Event Patterns Effectively
Design event patterns to filter events as precisely as possible to reduce the amount of unnecessary event processing. Avoid overly broad patterns that result in excessive event traffic.
3. Secure Access to EventBridge
Use AWS Identity and Access Management (IAM) to control access to your EventBridge resources. Ensure that only authorized users and services can publish or subscribe to events.
4. Optimize Event Delivery
If you’re using Lambda as a target, consider using an EventBridge retry policy to ensure that failed events are retried.
5. Monitor Event Flow
Use AWS CloudWatch Logs and CloudWatch Metrics to monitor event flow and target processing. This helps in diagnosing any issues or performance bottlenecks.
Case Study: E-Commerce Order Processing
In an e-commerce system, EventBridge can be used to decouple the order processing flow. When a customer places an order, an event is generated and sent to EventBridge. Based on the event type, different services process the order, such as inventory management, payment gateway integration, and shipment tracking.
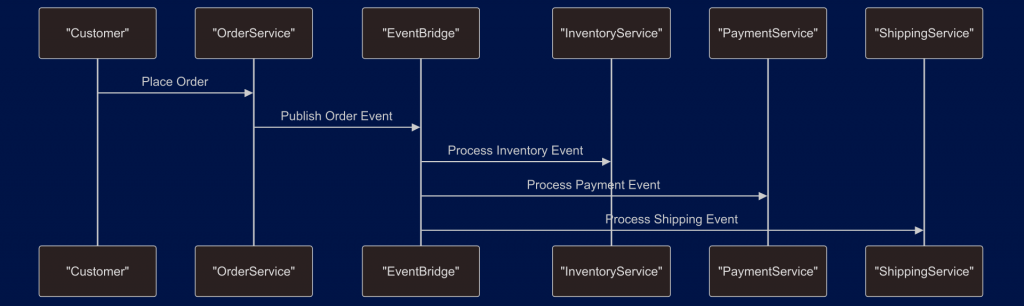
Future Outlook
As cloud technologies continue to evolve, we can expect the following trends related to event-driven architectures:
- Event-Driven Microservices: EventBridge will play an increasingly important role in orchestrating communication between microservices.
- Integration with AI/ML: Events can trigger AI or machine learning workflows, enabling intelligent decision-making in real time.
- Hybrid and Multi-Cloud Architectures: EventBridge’s ability to integrate with external services will support more hybrid and multi-cloud event-driven systems.
Conclusion
AWS EventBridge is a powerful tool for building decoupled, event-driven systems. By leveraging its ability to publish, route, and process events, developers can create highly scalable and resilient architectures. When implemented correctly, EventBridge enables businesses to build systems that are flexible, fault-tolerant, and easy to extend.
In modern software development, event-driven architectures (EDA) have gained immense popularity for their ability to create scalable, flexible, and resilient systems. One of the key challenges in building such systems is maintaining a decoupled communication model between different components. AWS EventBridge is a serverless event bus service that plays a crucial role in achieving this decoupling by facilitating the seamless transfer of events between services in real-time.