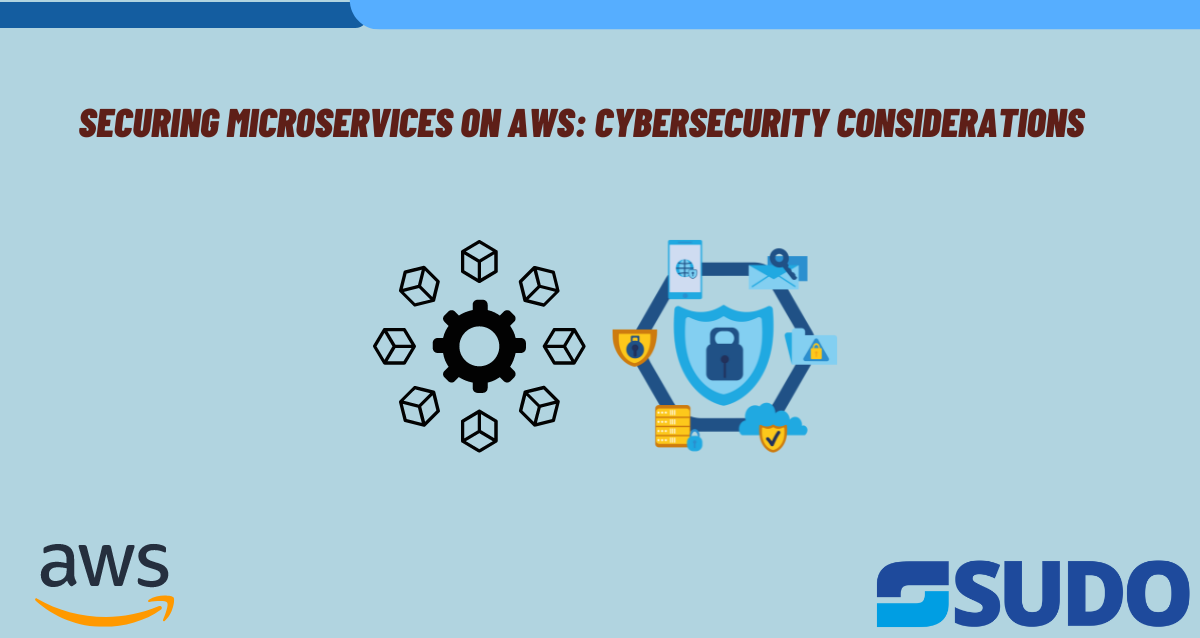
Introduction
Overview of Microservices Architecture
Microservices architecture is a design approach where applications are structured as a collection of loosely coupled, independently deployable services. Each service corresponds to a specific business functionality and communicates with other services through well-defined APIs. This architecture provides numerous benefits, such as scalability, ease of deployment, and the ability to use different technologies for different services. However, it also introduces unique security challenges due to the distributed nature of the services and the increased surface area for potential attacks.
Importance of Cybersecurity in Microservices
Given the distributed nature of microservices, security becomes a critical concern. Each microservice could be a potential entry point for attackers, making it essential to secure every service and the communication between them. Common threats include unauthorized access, data breaches, and man-in-the-middle attacks, all of which can be mitigated with proper security measures. Therefore, robust cybersecurity practices must be integrated into the design and deployment of microservices.
Purpose of the Article
This article aims to provide a comprehensive guide to securing microservices on AWS, focusing on both AWS CLI and Management Console steps. By following this guide, you will learn how to implement security best practices, including network isolation, identity and access management, encryption, monitoring, and incident response.
Security by Design: Architecting Secure Microservices
Principle of Least Privilege (PoLP)
The Principle of Least Privilege (PoLP) is a fundamental security concept that dictates that each component of the system should have the minimum level of access necessary to perform its function. In the context of microservices, this means that services should only have the permissions required to access specific resources.
Hands-on: Creating an IAM Policy with Least Privilege Using CLI
Create a custom IAM policy that grants specific permissions:
aws iam create-policy --policy-name MyMicroservicePolicy --policy-document file://microservice-policy.json
Example microservice-policy.json
:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::my-bucket/*"
}
]
}
Attach the policy to an IAM role:
aws iam create-role --role-name MyMicroserviceRole --assume-role-policy-document file://trust-policy.json
aws iam attach-role-policy --role-name MyMicroserviceRole --policy-arn arn:aws:iam::aws:policy/MyMicroservicePolicy
Example trust-policy.json
:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Principal": {
"Service": "ecs-tasks.amazonaws.com"
},
"Action": "sts:AssumeRole"
}
]
}
Assign the role to an ECS task: Use the AWS Management Console or CLI to assign this role to your ECS task.
Network Isolation
Network isolation is critical in securing microservices, as it limits the ability of attackers to move laterally within your environment.
Hands-on: Setting Up VPCs, Subnets, and Security Groups
Create a VPC:
aws ec2 create-vpc --cidr-block 10.0.0.0/16
Create subnets:
aws ec2 create-subnet --vpc-id vpc-xxxxxxx --cidr-block 10.0.1.0/24
aws ec2 create-subnet --vpc-id vpc-xxxxxxx --cidr-block 10.0.2.0/24
Create security groups:
aws ec2 create-security-group --group-name MyMicroserviceSG --description "Security group for microservices" --vpc-id vpc-xxxxxxx
Set up inbound and outbound rules:
aws ec2 authorize-security-group-ingress --group-id sg-xxxxxxx --protocol tcp --port 80 --cidr 0.0.0.0/0
aws ec2 authorize-security-group-egress --group-id sg-xxxxxxx --protocol tcp --port 443 --cidr 0.0.0.0/0
Attach the security groups to ECS/EKS services via the AWS Management Console or using the CLI.
Microservices Communication Security
Securing communication between microservices is crucial, especially in a distributed environment where services may communicate over public networks.
Hands-on: Implementing TLS for Microservices Communication
Create an ACM certificate:
aws acm request-certificate --domain-name my-service.example.com --validation-method DNS
Validate the certificate: Use the DNS validation method provided by AWS ACM.
Configure NGINX/Envoy Proxy to enforce TLS: Use the following example for NGINX:
server {
listen 443 ssl;
server_name my-service.example.com;
ssl_certificate /etc/nginx/ssl/my-service.example.com.crt;
ssl_certificate_key /etc/nginx/ssl/my-service.example.com.key;
location / {
proxy_pass http://my_microservice;
}
}
Restart NGINX:
sudo systemctl restart nginx
Optional: Enabling mTLS Using AWS App Mesh
Create a Mesh:
aws appmesh create-mesh --mesh-name my-mesh
Enable mTLS within the mesh: Configure the virtual gateway and virtual nodes to enforce mTLS using the console or AWS CLI.
Identity and Access Management (IAM) in Microservices
Role-based Access Control (RBAC)
RBAC is essential for defining access control policies based on roles assigned to users or services, ensuring that only authorized entities can access specific resources.
Hands-on: Implementing RBAC in Kubernetes (EKS)
Create a Kubernetes role:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: microservice-role
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
Bind the role to a service account:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: microservice-rolebinding
namespace: default
subjects:
- kind: ServiceAccount
name: microservice-sa
namespace: default
roleRef:
kind: Role
name: microservice-role
apiGroup: rbac.authorization.k8s.io
Apply the configuration:
kubectl apply -f role.yaml
kubectl apply -f rolebinding.yaml
Service-to-Service Authentication and Authorization
Hands-on: Implementing IAM Roles for Service Accounts in EKS
Create an IAM OIDC provider:
eksctl utils associate-iam-oidc-provider --cluster my-cluster --approve
Create an IAM role for the service account:
aws iam create-role --role-name EKSServiceRole --assume-role-policy-document file://trust-policy.json
Attach policies to the role:
aws iam attach-role-policy --role-name EKSServiceRole --policy-arn arn:aws:iam::aws:policy/AmazonS3ReadOnlyAccess
Associate the role with a service account:
apiVersion: v1
kind: ServiceAccount
metadata:
name: my-service-account
annotations:
eks.amazonaws.com/role-arn: arn:aws:iam::123456789012:role/EKSServiceRole
kubectl apply -f service-account.yaml
Hands-on: Implementing AWS STS for Temporary Credentials
Generate temporary credentials:
aws sts assume-role --role-arn arn:aws:iam::123456789012:role/RoleName --role-session-name MySession
Use the temporary credentials in your microservice to access AWS resources securely.
Data Protection and Encryption
Encryption at Rest
Encrypting data at rest ensures that even if storage devices are compromised, the data remains secure.
Hands-on: Enabling EBS, RDS, and S3 Encryption
Encrypt a new EBS volume:
aws ec2 create-volume --size 100 --region us-west-2 --availability-zone us-west-2a --volume-type gp2 --encrypted
Enable encryption on an RDS instance:
aws rds modify-db-instance --db-instance-identifier mydbinstance --storage-encrypted
Enable default encryption for an S3 bucket:
aws s3api put-bucket-encryption --bucket my-bucket --server-side-encryption-configuration '{
"Rules": [{
"ApplyServerSideEncryptionByDefault": {
"SSEAlgorithm": "AES256"
}
}]
}'
Use AWS KMS for key management:
Create a KMS key:
aws kms create-key --description "KMS key for microservices"
Encryption in Transit
Encryption in transit protects data as it moves across networks, ensuring that it cannot be intercepted or tampered with.
Hands-on: Implementing HTTPS Using ACM Certificates
Request an ACM certificate:
aws acm request-certificate --domain-name my-service.example.com --validation-method DNS
Validate the certificate via the AWS Management Console.
Attach the certificate to an API Gateway or Load Balancer: Use the Console to select the certificate when setting up HTTPS listeners.
Optional: Using AWS Secrets Manager for Managing Sensitive Data
Store a secret:
aws secretsmanager create-secret --name MySecret --secret-string "MySuperSecretPassword"
Retrieve a secret:
aws secretsmanager get-secret-value --secret-id MySecret
Monitoring, Logging, and Incident Response
Continuous Monitoring and Logging
Continuous monitoring and logging provide visibility into the activities within your microservices, enabling you to detect and respond to suspicious activities.
Hands-on: Setting Up CloudWatch Logs and CloudTrail for Audit Trails
Create a CloudWatch log group:
aws logs create-log-group --log-group-name MyMicroserviceLogs
Set up a retention policy:
bashCopy codeaws logs put-retention-policy --log-group-name MyMicroserviceLogs --retention-in-days 7
Enable CloudTrail:
aws cloudtrail create-trail --name MyTrail --s3-bucket-name my-bucket
aws cloudtrail start-logging --name MyTrail
Monitor logs using the CloudWatch console or CLI:
aws logs filter-log-events --log-group-name MyMicroserviceLogs --filter-pattern "ERROR"
Security Incident Response
A proactive incident response plan ensures that security incidents are handled swiftly and effectively, minimizing potential damage.
Hands-on: Configuring AWS Config Rules and GuardDuty
Enable AWS Config:
aws configservice put-configuration-recorder --configuration-recorder name=default --role-arn arn:aws:iam::123456789012:role/config-role
Create custom Config rules:
aws configservice put-config-rule --config-rule file://config-rule.json
Example config-rule.json
:
{
"ConfigRuleName": "restricted-ssh",
"Source": {
"Owner": "AWS",
"SourceIdentifier": "INCOMING_SSH_DISABLED"
}
}
Enable GuardDuty:
aws guardduty create-detector --enable
Respond to incidents using Lambda and CloudWatch Events: Example of setting up a Lambda function triggered by specific CloudWatch Events (e.g., unauthorized access attempts).
Secure Deployment Strategies
CI/CD Pipeline Security
Securing the CI/CD pipeline prevents attackers from injecting malicious code into your microservices.
Hands-on: Implementing Security Checks in AWS CodePipeline
Create a CodePipeline:
aws codepipeline create-pipeline --cli-input-json file://pipeline.json
Integrate security scanning tools like AWS CodeGuru: Example configuration to add a security scanning stage in CodePipeline.
Automated Vulnerability Scanning
Automated scanning helps identify vulnerabilities in containers and applications before they are deployed.
Hands-on: Integrating ECR Image Scanning and Other Tools
Enable image scanning in ECR:
aws ecr put-image-scanning-configuration --repository-name my-repo --image-scanning-configuration scanOnPush=true
Set up notifications for vulnerabilities:
aws sns create-topic --name ECRScanFindings
aws ecr put-image-scanning-configuration --repository-name my-repo --image-scanning-configuration scanOnPush=true
Immutable Infrastructure and Deployment
Immutable infrastructure ensures that once a service is deployed, it cannot be modified, reducing the risk of security breaches.
Hands-on: Implementing Blue-Green or Canary Deployments in ECS/EKS
Set up Blue-Green deployment with CodeDeploy:
aws deploy create-deployment --application-name MyApp --deployment-group-name MyDG --deployment-config-name CodeDeployDefault.ECSAllAtOnce --task-definition MyTaskDef:1 --service-name MyService
Example of using AWS Fargate for immutable infrastructure: Deploy new versions of your services as new Fargate tasks, rather than modifying existing ones.
Advanced Security Considerations
Zero Trust Architecture (ZTA)
Zero Trust Architecture is a security model that assumes no part of the network is inherently trusted, enforcing strict access controls and continuous monitoring.
Hands-on: Implementing ZTA in an AWS Environment
- Enforce least privilege access using IAM policies as described earlier.
- Implement continuous monitoring using CloudWatch and AWS Config.
- Enable multi-factor authentication (MFA) for sensitive operations:
aws iam enable-mfa-device --user-name my-user --serial-number arn:aws:iam::123456789012:mfa/MyMFADevice --authentication-code-1 123456 --authentication-code-2 789012
Security for Serverless Microservices
Serverless microservices, such as those running on AWS Lambda, present unique security challenges due to their ephemeral nature.
Hands-on: Implementing Security Best Practices in AWS Lambda
Create a Lambda function with an IAM role that enforces least privilege:
aws lambda create-function --function-name MyFunction --runtime python3.8 --role arn:aws:iam::123456789012:role/MyLambdaRole --handler lambda_function.lambda_handler --zip-file fileb://function.zip
Encrypt environment variables:
aws lambda update-function-configuration --function-name MyFunction --environment Variables={KEY=Value} --kms-key-arn arn:aws:kms:us-west-2:123456789012:key/my-key
Secure API Gateway integrations: Use the AWS Management Console to configure API Gateway to require API keys and use HTTPS endpoints.
Securing Multi-Cloud Microservices
Securing microservices in a multi-cloud environment requires additional considerations, particularly around consistent security policies and secure communication between clouds.
Hands-on: Configuring Security Controls for Cross-Cloud Microservices Communication
Set up a Transit Gateway for secure communication:
aws ec2 create-transit-gateway --description "My Transit Gateway"
Configure VPC peering between AWS and other cloud providers to ensure secure communication channels.
Conclusion
In this article, we covered various security best practices for securing microservices on AWS, including network isolation, IAM, encryption, monitoring, incident response, and secure deployment strategies. As microservices continue to evolve, so will the security landscape. Emerging trends like Zero Trust Architecture and advanced monitoring tools will play an increasingly important role in securing microservices. Securing microservices is a continuous process that requires constant vigilance and adaptation to new threats. By following the best practices outlined in this article, you can significantly enhance the security posture of your microservices on AWS.