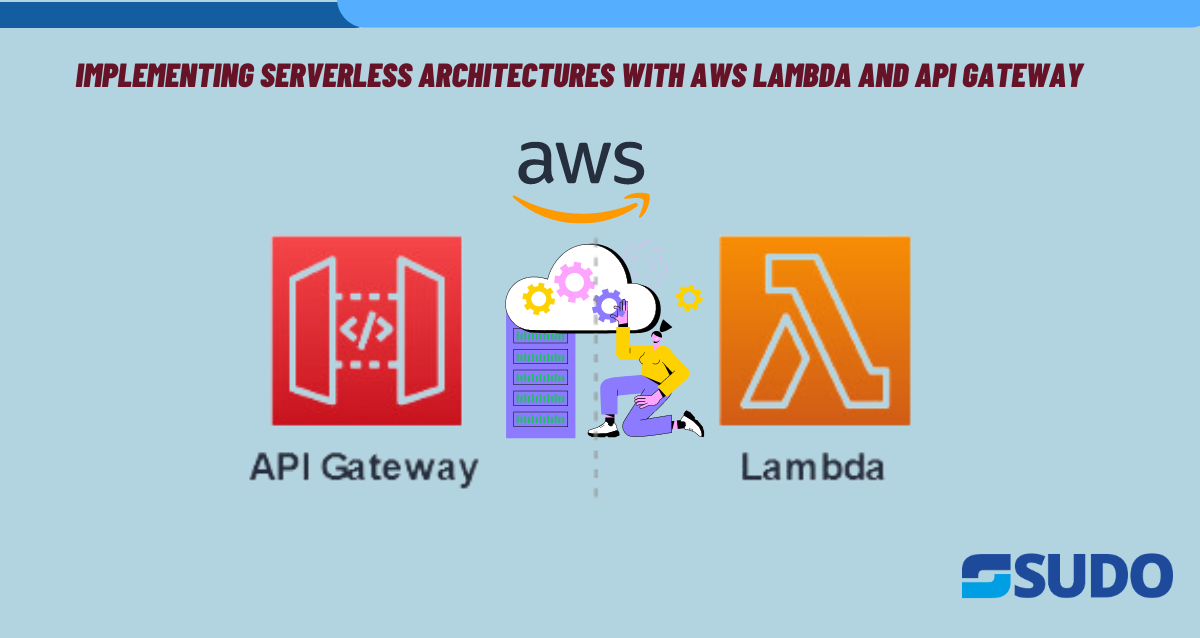
Introduction
What is Serverless Computing?
Serverless computing is a model in which the cloud provider manages the infrastructure required to run the applications. This includes the hardware, operating system, and runtime environment. With serverless computing, developers can focus on writing code without worrying about the underlying infrastructure.
Serverless architectures have become increasingly popular due to their scalability, reduced infrastructure management and cost-effectiveness. Among the tools for serverless computing in aws , Amazon Web Services (AWS) offers two key services for implementing serverless architectures:
- AWS Lambda
- Amazon API Gateway
In this article, we are going to walk through the process of building a serverless application using these services, with code, examples and AWS CLI commands.
Overview of AWS Lambda and API Gateway
AWS Lambda, a serverless computing service which allows you to run your code without provisioning or managing servers. You can build applications using Lambda functions triggered by events from various AWS services or custom sources.
Amazon API Gateway which is a fully managed service that allows you to create then publish, and manage APIs for your applications. You can use API Gateway to define RESTful or WebSocket APIs that enable your Lambda functions to be triggered by HTTP requests.
In this step-by-step guide we will create a serverless web application for a logistics system using AWS Lambda and API Gateway. We will create Lambda functions to handle different operations and use API Gateway to create a REST API to interact with these Lambda functions.
1. Set up your AWS account and environment
- Sign up for an AWS account if you don’t have one.
- Install and configure the AWS CLI (Command Line Interface).
- Set up the necessary IAM roles for Lambda and API Gateway.
2. Create Lambda functions for each operation
- Sign in to the AWS Management Console.
- Navigate to the Lambda service.
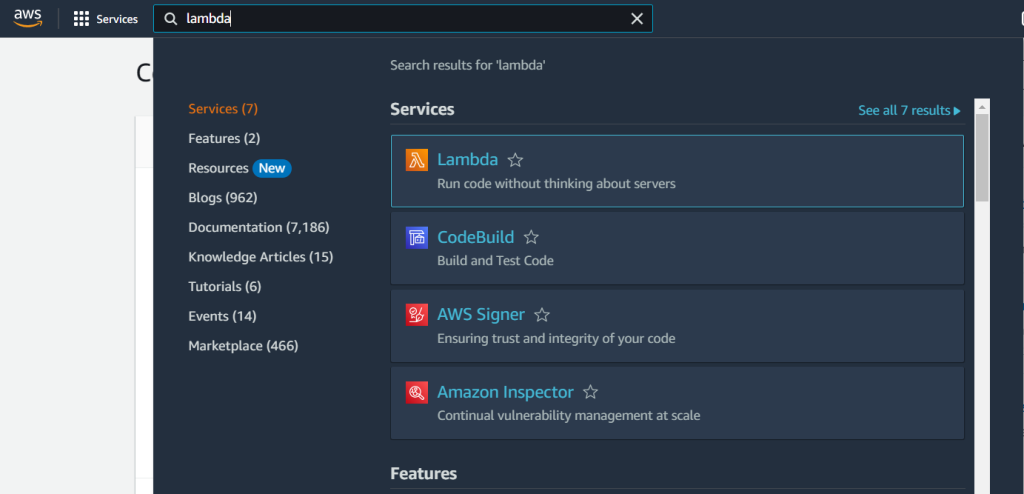
- Click “Create function”.
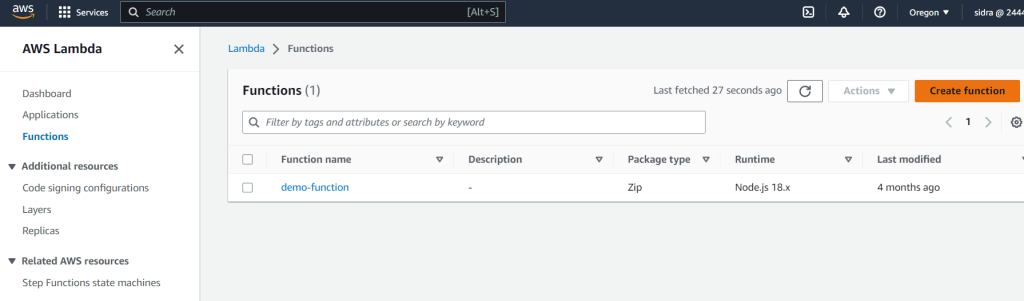
- Choose “Author from scratch”.
- Enter a name for your Lambda function (e.g., “SudoServerlessFunction”).
- Choose the desired runtime (e.g., Python, Node.js, or Java).
- Create or choose an existing IAM role with the necessary permissions.
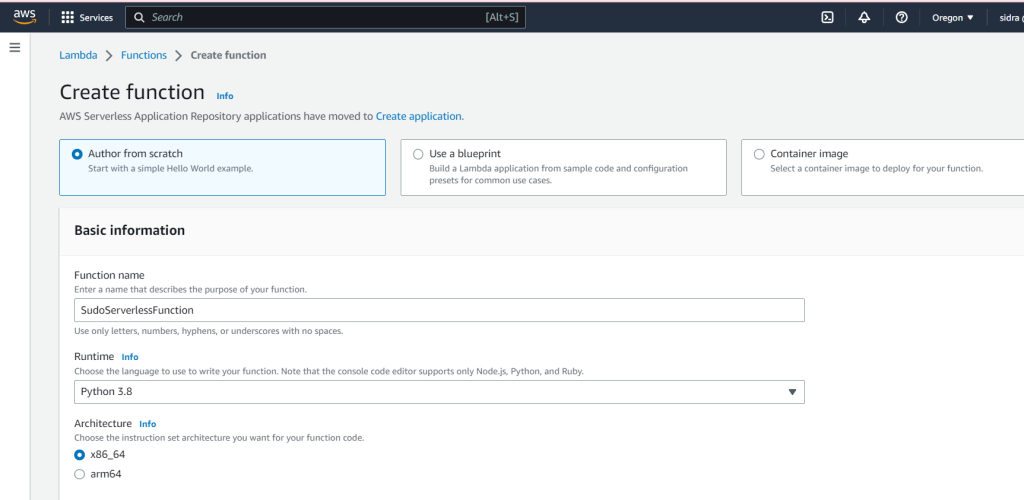
- Click “Create function”.
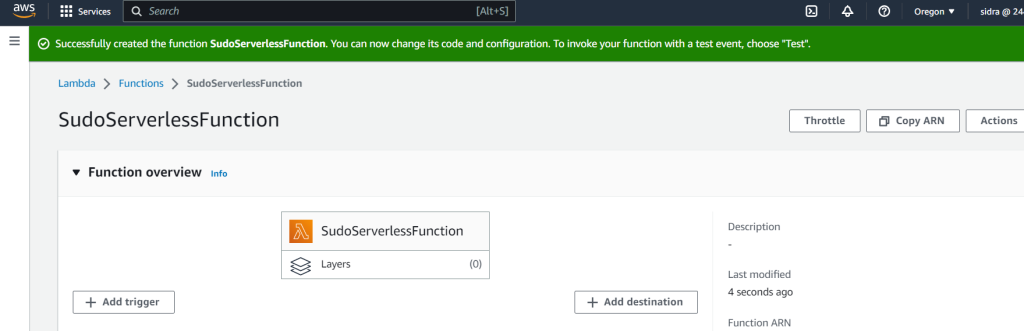
For example, create a Lambda function to handle the POST operation for adding a new shipment:
import json
def add_shipment(event, context):
body = json.loads(event['body'])
shipment_id = body['shipment_id']
destination = body['destination']
status = "Pending"
# Implement logic to store the shipment data, e.g., using DynamoDB
response = {
"statusCode": 200,
"body": json.dumps({
"message": f"Shipment {shipment_id} added.",
"shipment_id": shipment_id,
"destination": destination,
"status": status
})
}
return response
- In the “Function code” section, choose the “Upload a .zip file” or “Upload a file from Amazon S3” option.
- Upload your function code (ensure that your code follows the requirements for the chosen runtime).
- Set the “Handler” to the function entry point (e.g., for Python, it could be “Sudo_module.SudoServerlessFunction”).
Create the Lambda function using the AWS CLI:
aws lambda create-function --function-name AddShipment --runtime python3.8 --role <YOUR_LAMBDA_ROLE_ARN> --handler lambda_function.add_shipment --zip-file fileb://lambda_function.zip
Similarly, create Lambda functions for other operations like GET and PUT.
3. Configuring the Lambda Function
- In the “Designer” section, click on “Add trigger”.
- Select “API Gateway” as the trigger.
- Choose “Create a new API” or “Use an existing API”, depending on your requirements.
- Set the security settings for the API, such as “Open” or “AWS_IAM”.
- Click “Add”.
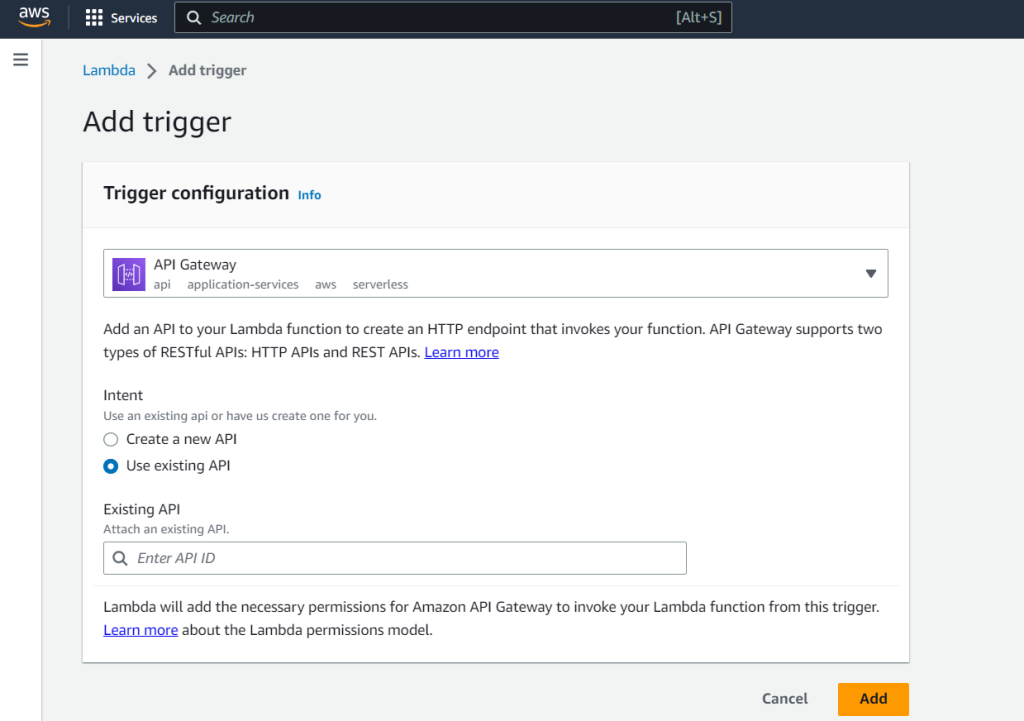
4. Configure API Gateway
- Navigate to the API Gateway service in the AWS Management Console.
- Click on your API.
- Define your resources and methods (e.g., GET, POST, PUT, DELETE).
- For each method, set the “Integration type” to “Lambda Function”.
- Choose the Lambda function you created earlier.
- Configure the mapping templates and request/response transformations, if necessary.
- In the “Actions” menu, click “Deploy API”.
- Choose a deployment stage, or create a new one.
- Note the “Invoke URL” provided for your API. This URL is used to call your API.
Example: Integrate the POST method with the AddShipment Lambda function using the AWS CLI:
aws apigateway put-integration --rest-api-id <YOUR_REST_API_ID> --resource-id <YOUR_RESOURCE_ID> --http-method POST --type AWS_PROXY --integration-http-method POST --uri arn:aws:apigateway:<REGION>:lambda:path/2023-03-31/functions/arn:aws:lambda:<REGION>:<ACCOUNT_ID>:function:AddShipment/invocations
5. Deploy your serverless application
- Deploy your REST API in API Gateway.
- Note the API’s base URL, which will be used to make requests.
5. Test and monitor your application
- Use tools like Postman or curl to test your API endpoints.
Example: Test the POST operation with curl:
curl -X POST -H "Content-Type: application/json" -d '{"shipment_id": "123", "destination": "New York"}' https://<YOUR_API_BASE_URL>/shipment
6. Conclusion and future benefits of serverless architectures
You can create, deploy, and scale serverless web applications with AWS Lambda and API Gateway.
By adopting serverless architectures, you can focus on your application’s logic and leave the underlying infrastructure management to AWS, resulting in cost savings, improved performance, and faster development cycles. You can now expand your application by adding more functionality to the Lambda function, customizing the API Gateway resources and methods, or integrating other AWS services to enhance your serverless architecture.